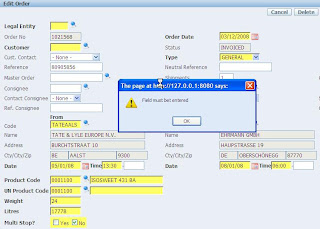
In APEX there is no property to mark a field as a required field (at this moment, could be an enhancement request!). However you can mark the prompt of a field by using the 'Required' template - with or without help. So why not use the APEX Repository to enhance the look-and-feel of your application and show the user which fields are required? And of course we use jQuery to accomplish this.
This is how you do it:
Create an On Demand Application Process (lets call it getRequiredItems) and fire it onLoad - After Header:
This process returns the items with a 'Required'-prompt for the current page in one long string, separated with a ~.
DECLARE
CURSOR c_items IS
SELECT item_name
FROM apex_application_page_items
WHERE item_label_template LIKE 'Required%'
AND page_id = :APP_PAGE_ID;
item_list varchar2(32000) := '';
item_sep varchar2(1) := '~';
BEGIN
FOR r_item IN c_items LOOP
item_list := item_list||r_item.item_name||item_sep;
END LOOP;
-- P0_REQUIRED_ITEMS is an hidden item on Page 0
:P0_REQUIRED_ITEMS := substr( item_list, 1, length( item_list ) -1);
END;
In Page 0, create a Javascript function that adds a class ('RequiredField') to the fields in the P0_REQUIRED_ITEMS string filled by the Application Process and (optional) adds an onblur event that calls a function (checkRequiredField) using
Now you can create an entry in your CSS file to give the required field a nice color (or whatever else you want).
function setRequiredItems(){
var colArray = $v("P0_REQUIRED_ITEMS").split("~");
for(i = 0; i < colArray.length; i++){
$('#'+colArray[i]).addClass("RequiredField").attr("onblur",'checkRequiredField(this)');
}
}
Next create the Javascript function to check if a required field is empty or not. If the field is empty, the user cannot navigate out of the field, unless he puts in a value.
function checkRequiredField(pThis){
if (!(pThis.value)){
alert('Field must be entered');
setTimeout(function(){pThis.focus();}, 5);
}
}
Now add this Javascript function to the jQuery document.ready function:
$(function(){
setRequiredItems();
});
And the result is something like the picture on the top of this post. A live example is available on apex.oracle.com.
Comments
Of course Apex's default templates add an * to each field with the Required label but this is much better, especially with the validation.
Now I'm not sure if I'd highlight the required fields that much, the yellow is a bit garish. But if your users need really obvious markers, so be it! :-)
Thanks.
nice tip!
Just a question, why don't you immediately generate the string with the items in the page 0 region? That would avoid the additional AJAX call to the server to get the items. I think that should be much faster and would save database resources.
Greetings
Patrick
Nice post! I know Patrick suggested that you make a Page 0 process to load the list. To help avoid the extra processing why not update the "required" template with a certain class and use jQuery to tag all the fields with that class as required. That way you wouldn't even need to run the process?
Martin.
Thanks for the update!
I messed up that page (trying something else), but it is working again. Check the value of P0_REQUIRED_ITEMS in Session State (and check that jQuery is loaded!).